In the previous tutorial we added a rotating shield to our player. The shield object would move in uniform circular speed around the player object. The shield was a kinematic body so that we can set velocity every frame to control its movement.
In this tutorial, we will learn how to add an explosion using BoundingBox (QueryAABB method). This will be part 1 of the explosion tutorial. In the next one we will refine the explosion using raycast method.
What is a BoundingBox
As the name suggests, Bounding Box is a rectangle which can cover the full shape of the body. It is useful in detecting collision between two objects. There are of two types: Oriented Bounding Box(OBB) and Axis Aligned Bounding Box. The difference between them is that the edges of OBB are not parallel to the basis vectors of the frame and edges of Axis Aligned Bounding Box have to parallel to the axis which would mostly be x axis and y axis for 2-dimensional games. For 3d games edges would be parallel to x,y and z axis. Axis Aligned Bounding Box takes less computational time to process collision detection but is less exact than OBB.
In Box2d, QueryAABB command, we are using an Axis Aligned Bounding Box. Lets take a look at the signature.
World.QueryAABB( QueryCallback callback, Vector2 bottomLeft, Vector2 topRight)
Its parameters are World.QueryAABB( QueryCallback callback, Vector2 bottomLeft, Vector2 topRight). QueryCallback is a callback class which has a reportFixture method and it is called whenever a fixture is falling inside the bounding box. BottomLeft and TopRight vectors define the two diagonal end points to define the shape of the rectangle. reportFixture returns a boolean so if we want to stop the query, we can return false. Below is a snippet to show QueryAABB in action.
QueryCallback queryCallback; Array<Body> bodies; public void Init() { .... callback=new QueryCallback() { @Override public boolean reportFixture(Fixture fixture) { //Add bodies to an array which we can process after the query is finished bodies.add(fixture.getBody()); //To keep on checking other fixtures, return true return true; } }; } public boolean touchUp(float screenX, float screenY, int pointer, int button){ .... bodies.clear(); //We are using a 1:100 ratio for box to our world coordinates float x=screenX*0.01f ; float y=screenY*0.01f ; float boxBlastRadius=2 ; //In world coordinates its 200 units world.QueryAABB(callback,x-boxBlastRadius,y-boxBlastRadius, x+boxBlastRadius,y+boxBlastRadius); //The above query to search for bodies runs synchronously, so when we go the next line in code, the list of bodies are computed which are present in the above bounding box return false; ... }
In the above code, first we are initiallizing the callback. reportFixture is being called when query encounters a fixture in bounding box. We are adding it to the array so we can draw points at their position for debugging. Then in touchUp (event after mouse click), we are capturing the location of click and creating a bounding box of 400 by 400 pixels. Below is an image for reference
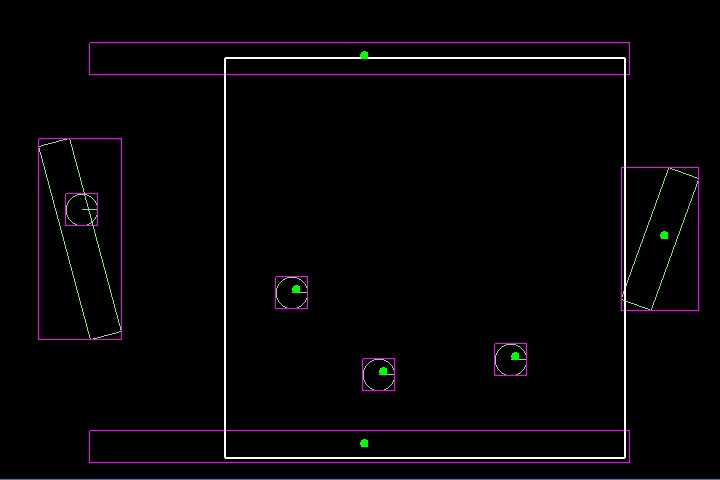
Next Step?
Here we are able to get list of bodies which are present in blast radius. There is a issue though with this solution. The blast radius is in rectangle shape rather than a circular shape. So the world bodies near the vertices of this bounding box might be outside the circular blast radius. To solve this problem we can make use of raycast method in box2d which we will cover in next tutorial.
Below is link to github code repository which has an example of QueryAABB along with other examples.