Hi,
In this small post we would write on how to show android dialogs in libgdx code. It is pretty simple. We used dialogs when we had some processing going on or while changing screens.
Create A Dialog:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" > <ImageView android:id="@+id/imageWaitDlg" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/pleasewait" android:contentDescription="@string/waitString" /> </LinearLayout>
Above is a sample xml for a dialog. android:src is pointing to an image which is located in the resources/drawable directory of the project. Below is the code to initialize the dialog which can be called in OnCreate function or anytime after that.
Dialog waitDialog; private void InitDialog() { waitDialog = new Dialog(this); waitDialog.requestWindowFeature(Window.FEATURE_NO_TITLE); waitDialog.setContentView(R.layout.dialog); waitDialog.getWindow().setBackgroundDrawable(new ColorDrawable(0)); }
Important Points:
- We did not want any space for title in the dialog so the following line is there :-waitDialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
- We wanted a transparent background for the dialog which can be achieved by the following line of code :- waitDialog.getWindow().setBackgroundDrawable(new ColorDrawable(0));
public Interface WaitDialogInterface{ public void ShowDialog(); public void HideDialog(); }
Implement the Interface:
public class AndroidGame extends AndroidApplication implements WaitDialogInterface{ ....... Handler dialogHandler; private void InitDialog(){ dialogHandler=new Handler(); waitDialog = new Dialog(this); waitDialog.requestWindowFeature(Window.FEATURE_NO_TITLE); waitDialog.setContentView(R.layout.dialog); waitDialog.getWindow().setBackgroundDrawable(new ColorDrawable(0)); } public void ShowDialog(){ dialogHandler.post(showDialogRunnable); } final Runnable showDialogRunnable=new Runnable(){ @Override public void run() { // TODO Auto-generated method stub if(waitDialog!=null && !waitDialog.isShowing()) waitDialog.show(); } }; public void HideDialog(){ dialogHandler.post(hideDialogRunnable); } final Runnable hideDialogRunnable=new Runnable(){ @Override public void run() { // TODO Auto-generated method stub if(waitDialog!=null && !waitDialog.isShowing()) waitDialog.dismiss(); } }; }
Note:
We need to attach a Runnable object because we are not calling these from the UI thread so we would get an error. So we create a handler so that the messages posted by it becomes part of the UI thread.
Call the functions from Game Code:
Now finally we just need to call these dialogs to show up in the game. For eg when you are disposing a screen and going to another screen then it can take sometime so call ShowDialog() in dispose function of a screen and call HideDialog() in the init function of the other screen.
SourceCode:
In the source code attached there is a “show dialog” image, which on clicking would show the dialog and on back press the dialog would disappear. Click on the image below to download. Rar contains eclipse project which can be imported into the workspace.
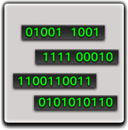
Thanks. If you have any queries/suggestions please post them in comments.
your tutorials are of a great help,thanks alot! i searched the entire net and no one has made such awesome yet simple to understand tutorials
thanks again
hi can you add source code for this tutorial?
Hi,
We just added source code for this tutorial. Hope it helps.
Thanks
Thank you very much!
Thank you for this great tutorial, can you make tutorial how to implement WebView in libgdx?